Are you tired of manually manipulating arrays and strings in your JavaScript code? Look no further than the powerful JavaScript shift() method! With a few lines of code, you can transform your data structures with unparalleled efficiency.
In this blog post, we will dive deep into:
- The shift() method in JavaScript
- How it can be used to manipulate arrays and strings
- Compare it to other similar methods like pop() and unshift()
- How it differs in terms of time complexity
What is sift() JavaScript array method?
The shift() is one of the built-in JavaScript array methods. Which is used to remove the first element of an array. It will modify the original array, reduce the size, and return the removed element. If the array is empty, the shift()
method will return "undefined"
and the array is not modified.
The syntax for the shift()
method is:
arrayName.shift()
Here, arrayName
is the name of the array. The shift method removes the first element from an array and returns it.
JavaScript shift() array elements
JavaScript array
is one of the non-primitive data types. Arrays are collections of elements. It can contain any type of data, such as numbers
, strings
, objects
, etc.
Let’s take a look at how to use the shift()
in the JavaScript method on the array with an example:
const evenNumbers = [2, 4, 6, 8, 10];
const shiftedNumber = evenNumbers.shift();
console.log(evenNumbers);
// Output: [4, 6, 8, 10]
console.log(shiftedNumber);
// Output: 2
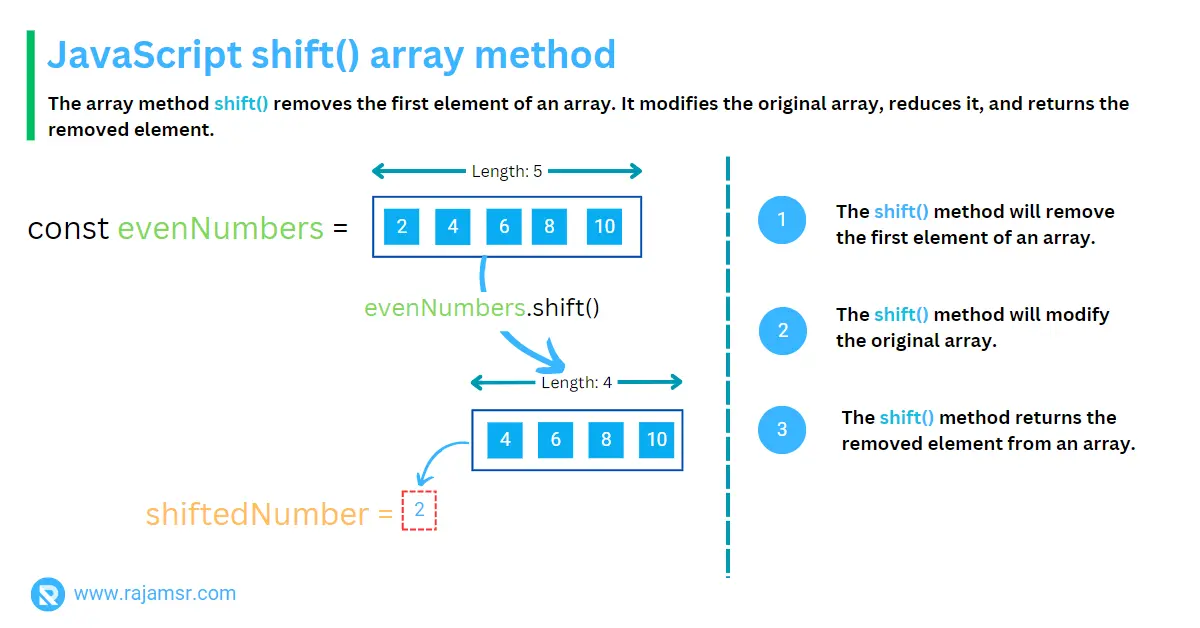
In the above example, we have an array of even numbers. Using the array shift()
JavaScript method, the first element is removed from the array; in this case, it is value 2
. The removed number 2
is assigned to the JavaScript variable shiftedNumber
. We then log the modified array and the removed element.
Comparison of shift() vs pop()
JavaScript has a built-in array method called pop()
. Which will remove the last element of an array. The shift()
JavaScript method removes the first element, while the pop()
method removes the last element in JavaScript arrays.
Here’s an example of the shirt()
and pop()
methods:
const evenNumbers = [2, 4, 6, 8, 10];
const poppedNumber = evenNumbers.pop();
console.log(evenNumbers);
// Output: [2, 4, 6, 8]
console.log(poppedNumber);
// Output: 10
There are several ways to use JavaScript array clear to empty the entire array.
Comparison of shift() vs unshift()
JavaScript has a built-in array method called unshift()
. The JavaScript unshift() method adds elements to the beginning of an array. In contrast, the shift()
JavaScript method removes the first element.
Here’s an example of the unshift()
method:
let array = ['Yes', 'No'];
array.unshift('Select');
console.log(array);
// Output: ["Select", "Yes", "No"]
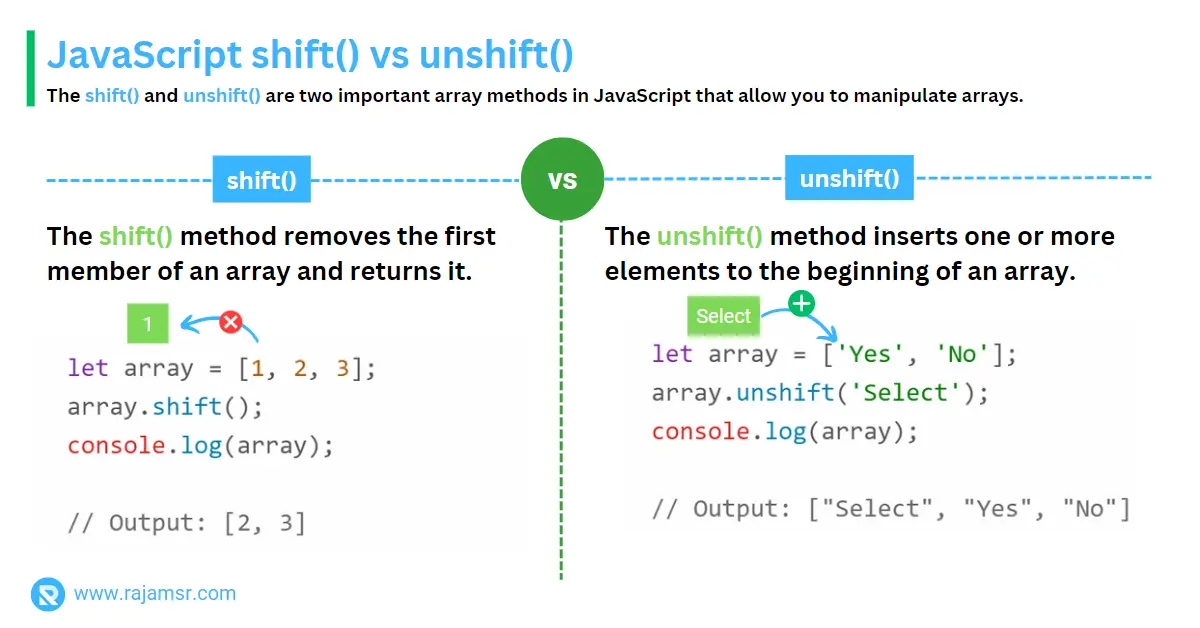
The shift() method on string
However, you cannot use the shift()
method to remove the first character of a JavaScript string. It will throw an "shift is not a function"
error.
Here’s an example:
let greet = "Hello World!";
const shiftedChar = greet.shift();
// Output: greet.shift is not a function
You can easily fix it by using the split()
method. The string split() method will convert a string into an array of characters. Once converted as an array, you can apply the shift()
method to remove the first characters.
Here’s an example of how to use the shift()
method to remove the first characters from the string:
let greet = "Hello JavaScript!";
const shiftedChar = greet.split('').shift();
console.log(shiftedChar);
// Output: "H"
Shift() elements in array using iteration
To shift every element of an array in JavaScript, we can iterate over each element using a for loop and apply the shift() method to each element. Here’s an example:
const colors = ['red', 'green', 'blue'];
while (colors.length > 0) {
const shiftedNumber = colors.shift();
console.log(shiftedNumber);
}
// Output:
"red"
"green"
"blue"
In the above example, we have an array of numbers, and we’re using a for loop to iterate over each element. Inside the loop, we’re using the shift()
method to remove the first element of the array and log it to the console.
JavaScript array shift() time complexity
Internally, the shift()
method needs to shift all the elements to the left after removing the first element. This means that for an array with n elements, it will need to perform n-1
shifts.
Each shift()
operation involves copying the elements to a new position in memory, which takes a linear amount of time. As a result, the time complexity of shift()
is O(n)
, where n is the length of the array.
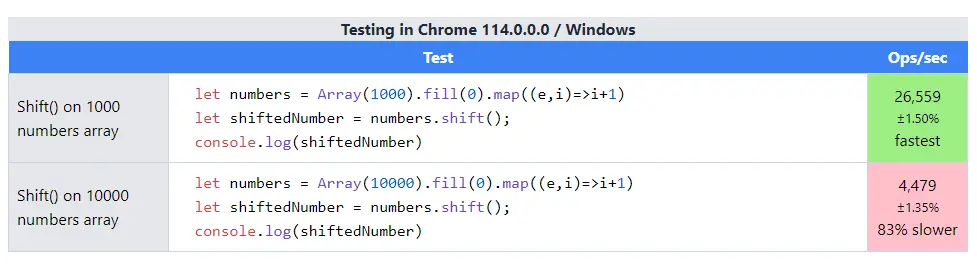
Time complexity is an important factor to consider when working with a large amount of data. The JavaScript shift time complexity is O(n), where n is the number of elements in the array. This means that for an array with n elements, the time taken to shift an element is proportional to n.
From the above screenshot, you can see that on the 1000-number array
, we could do 26559 shift() operations
per second. At the same time10,000-number array
, we could do only 4479 shift() operations
per second.
Conclusion
In this blog post, we’ve learned about the JavaScript shift()
method and how it can be used to manipulate arrays and strings. We’ve also compared it to other similar methods pop()
and unshift()
and see how it differs in terms of time complexity.
Finally, we’ve seen how we can use a for loop to iterate over an array and apply the shift()
method to each element.
By understanding the shift()
method in JavaScript, you can write more efficient and optimized code in your JavaScript applications.