Have you ever wondered how to JavaScript remove character from string? If you are working with text data in JavaScript, you may encounter situations where you need to modify or manipulate strings in various ways. For example, you may want to remove whitespace, punctuation, special characters, or certain letters from a string. How can you do that in JavaScript?
In this blog post, I will show you:
- How to remove string characters
- Removing a character at a given index
- Using regex to remove characters
- Performing removals asynchronously
- Conditional character removal
- Keeping only numbers in a string
- Removing characters on click event
- Removing characters one by one
- setInterval() for real-time removal
- Removing characters after a certain point
- Storing removed characters in a new variable
By the end of this post, you will be able to write clean and efficient code to manipulate strings in JavaScript.
1. JavaScript remove character from string
Whether you’re cleaning user input or formatting data, knowing how to remove characters from a JavaScript string is a valuable skill.
Let’s see how to remove a character from a string:
const originalString = "Hello, World!";
const removedCharacter = originalString.substring(0, 5) + " " + originalString.substring(7);
console.log(removedCharacter);
// Output: "Hello World!"
originalString
.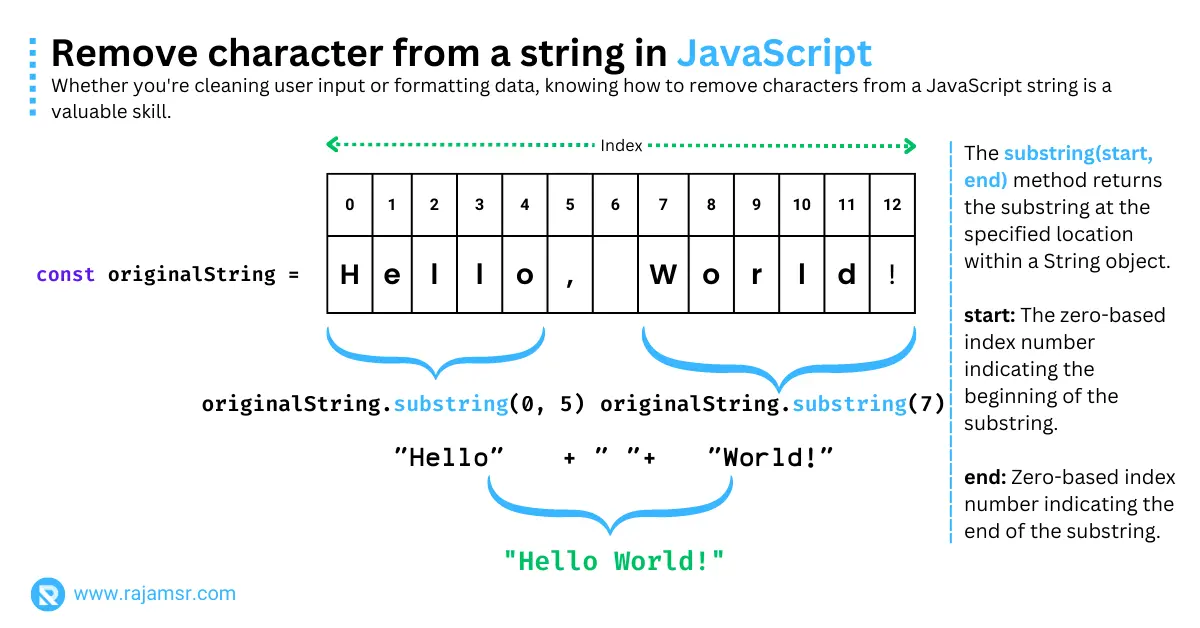
Alternatively, you can use the string replace()
method to remove characters from a string. The following method will replace a comma with an empty string.
const originalString = "Hello, World!";
const removedCharacter = originalString.replace(",","");
console.log(removedCharacter);
// Output: "Hello World!"
With this approach you can remove specific character from string in JavaScript.
2. JavaScript remove char from string at index
If you want to remove a character at a specific index, you can manipulate the string using indexing and JavaScript string concatenation. In this example, let’s see how to remove char from a string in JavaScript at a specific index.
function removeCharacterAtIndex(inputString, index) {
return inputString.slice(0, index) + inputString.slice(index + 1);
}
const originalString = "JavaaScript";
const indexToRemove = 4;
const modifiedString = removeCharacterAtIndex(originalString, indexToRemove);
console.log(modifiedString);
// Output: "JavaScript"
removeCharacterAtIndex()
function removes the character at the specified index from the input string using the JavaScript slice() method. This JavaScript function takes a string inputString
and an index, then creates a new string by removing the character at that index.3. Remove letters using regular expressions
Regular expressions offer a powerful way to remove characters based on patterns. Let’s see from the string how to remove characters using regex.
const stringWithNumbers = "Th1s is a str1ng w1th numb3rs";
const removedNumbers = stringWithNumbers.replace(/[0-9]/g, "");
console.log(removedNumbers);
// Output: "Ths is a strng wth numbrs"
The replace()
method with the regex [0-9]
matches and removes all numeric digits from the string.
4. Asynchronous removal operations
In scenarios requiring asynchronous character removal, you can utilize setTimeout()
for delayed execution.
function removeCharacterAsync(inputString, charToRemove, delay) {
setTimeout(() => {
const modifiedString = inputString.replace(charToRemove, "");
console.log(modifiedString);
}, delay);
}
removeCharacterAsync("Remove after a delay!", "!", 1000);
// Output (after 1 second): "Remove after a delay"
The removeCharacterAsync()
function asynchronously removes the specified character after the provided delay using setTimeout()
.
5. Remove character from string only if exists
If you wish to remove a character only if it exists in the string, you can use conditional statements. Here is an example of deleting a character from a string only if it exists in a string.
function removeCharIfExists(inputString, charToRemove) {
if (inputString.includes(charToRemove)) {
return inputString.replace(charToRemove, "");
}
return inputString;
}
const originalText = "Hello, JavaScript!";
const charToRemove = "!";
const modifiedText = removeCharIfExists(originalText, charToRemove);
console.log(modifiedText);
// Output: "Hello, JavaScript"
The removeCharIfExists()
function checks if the character "!"
exists before deleting it using the replace()
method. JavaScript includes() method that validates whether the character exists in a string.
6. Remove letters from string and leave number
When you need to retain numeric digits while removing characters, regular expressions can come to the rescue.
const mixedString = "abc12xyz3";
const onlyNumbers = mixedString.replace(/[^0-9]/g, "");
console.log(onlyNumbers);
// Output: "123"
The regex /[^0-9]/g
matches non-numeric characters, effectively removing certain characters from a string. To remove numbers from a string you change the regex pattern as shown in the following example:
const mixedString = "abc12xyz3";
const onlyChars = mixedString.replace(/[^a-zA-Z]/g, "");
console.log(onlyChars);
// Output: "abcxyz"
7. Delete character from string on button click
Interactive character removal can be achieved by triggering actions based on user interactions. Let’s explore removing characters on button clicks.
<!DOCTYPE html>
<html>
<head>
<title>Interactive Character Removal</title>
</head>
<body>
<input type="text" id="inputField" value="Hello, World!">
<button id="removeButton">Remove Comma</button>
<p id="result"></p>
<script>
const inputField = document.getElementById("inputField");
const removeButton = document.getElementById("removeButton");
const result = document.getElementById("result");
removeButton.addEventListener("click", () => {
const inputValue = inputField.value;
const modifiedValue = inputValue.replace(",", "");
result.textContent = modifiedValue;
});
</script>
</body>
</html>
In this example, you have an input field and a button. When the button is clicked, the comma in the input’s value is removed, and the result is displayed.
8. Remove characters from string each one by one
Iterative removal of characters can be achieved using loops. Let’s explore removing characters one by one using a loop.
function removeCharactersOneByOne(inputString, charToRemove) {
let result = inputString;
while (result.includes(charToRemove)) {
result = result.replace(charToRemove, "");
}
return result;
}
const originalText = "Mississippi";
const charToRemove = "i";
const modifiedText = removeCharactersOneByOne(originalText, charToRemove);
console.log(modifiedText);
// Output: "Msssspp"
The removeCharactersOneByOne()
function iterates and removes the specified character from the string until it’s no longer present.
However, if you don’t want to use while-loop
and replace all character instances, then you can use the string replaceAll()
method. The following code example also produces the same result.
const originalText = "Mississippi";
const charToRemove = "i";
const modifiedText = originalText.replaceAll(charToRemove, "");
console.log(modifiedText);
// Output: "Msssspp"
9. Real-time character removal using setInterval()
setInterval()
. This is particularly useful for dynamic content updates.let text = "Countdown: 5 seconds";
const interval = setInterval(() => {
text = text.replace(/\d+/, (match) => match - 1);
console.log(text);
if (text === "Countdown: 0 seconds") {
clearInterval(interval);
}
}, 1000);
In this example, we use setInterval()
to decrement the numeric value in the string every second, creating a countdown effect.
10. Removing characters after a specific character
In more complex scenarios, you might need to remove characters based on specific formats or positions within the string.
const email = "john.doe@domain.com";
const username = email.substring(0, email.indexOf('@'));
console.log(username);
// Output: "john.doe"
In this example, we remove characters after the @
symbol using substring()
and JavaScript indexOf(). Alternatively, you can use the JavaScript split()
method to remove a string after a specific character.
const email = "john.doe@domain.com";
const modifiedText = email.split('@')[0];
console.log(modifiedText);
// Output: "john.doe"
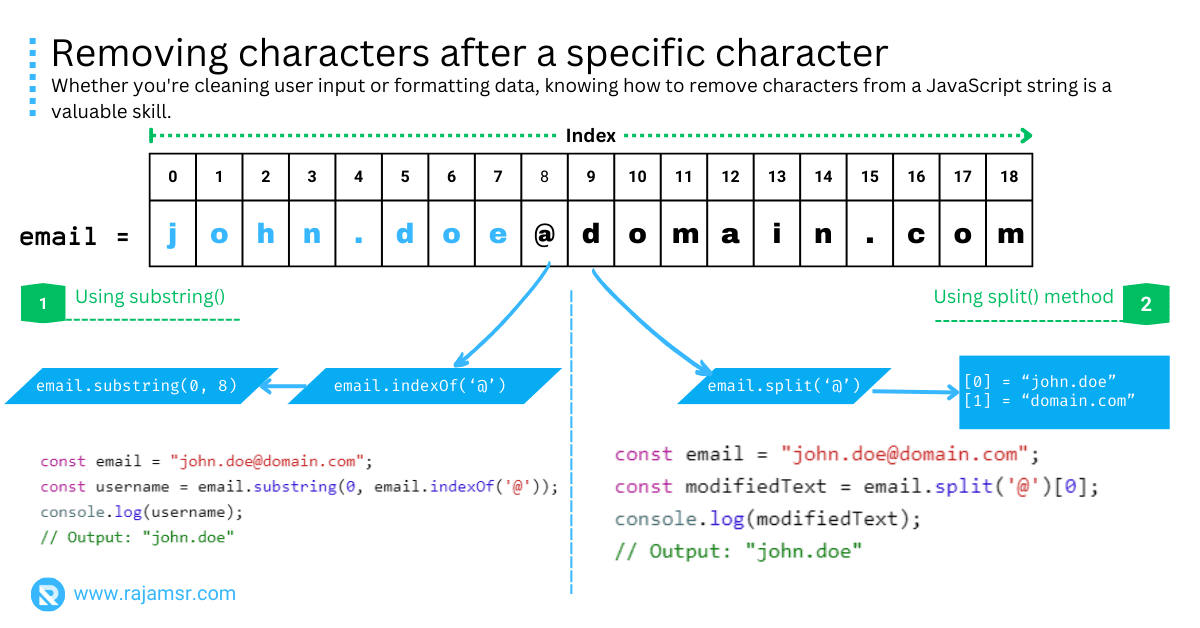
11. Remove character from string and hold in new variable
To ensure you preserve the original string while making modifications, store the modified string in a new JavaScript variable.
const originalString = "Hello, World!";
const modifiedString = originalString.replace(",", "");
console.log(originalString);
// Output: "Hello, World!"
console.log(modifiedString);
// Output: "Hello World!"
By assigning the result of replace()
to modifiedString
, the original string remains unaltered.
Frequently asked questions
You can chain the replace()
method to remove multiple characters.
let greeting = "Hello, World!"; greeting = greeting.replace(/o/g, '').replace(/l/g, ''); // Removes 'o' and 'l' console.log(greeting) // Output: He, Wrd!
You can use the string slice()
method to remove the first character. The following example code removes the first character comma from the string.
let greeting = ",Hello, World!"; greeting = greeting.slice(1, greeting.length); console.log(greeting) // Output: "Hello, World!"
You can use the slice()
method to remove the last character. The following code removes the last character "!"
from the string.
let greeting = "Hello, World!"; greeting = greeting.slice(0, -1); console.log(greeting) // Output: Hello, World
regular expression
to remove all digits.let greeting = "Hello, 123World!"; greeting = greeting.replace(/\d/g, ''); // Removes all digits console.log(greeting) // Output: Hello, World!
replace()
method to remove all spaces.let greeting = " Hello , World! "; // Option 1: greeting = greeting.replace(/\s/g, ''); console.log(greeting) // Output: "Hello,World!" // Option 2: greeting = greeting.replace(' ', ''); console.log(greeting) // Output: "Hello,World!"
const message=`Hello, "JavaScript`; console.log(message.replace('"',"")); // Output: "Hello, JavaScript"
Conclusion
I hope you enjoyed this comprehensive guide on how to remove characters from strings using JavaScript. I covered a range of methods, from simple ones like substring()
and slice()
to more complex ones involving regular expressions and asynchronous operations.
These methods will help you manipulate strings in various scenarios, whether you’re creating interactive user interfaces or processing data.
By mastering these techniques, you’ll be able to write efficient and effective JavaScript code.