Have you ever wondered how to check if a JavaScript array contains a certain value or element? This is a common problem that many developers face when working with arrays, especially when dealing with dynamic data or user input. You might want to perform some action based on whether an array includes or excludes a specific value, such as filtering, validating, or displaying data.
Fortunately, there is a simple and elegant solution to this problem: the Array.prototype.indexOf() method. This method returns a boolean value indicating whether an array contains a given value as an element. It is part of the ECMAScript 2016 standard and is widely supported by modern browsers.
In this blog post, I will show you:
- Searching for a string in a JavaScript array
- Finding an object within a JavaScript array
- Determining whether a value exists in a JavaScript array
- Looking for a key in a JavaScript array
- Checking for a boolean in a JavaScript array
- Matching a key-value pair in a JavaScript array
- Comparing a JavaScript array with another array
By the end of this post, you will be able to confidently use the JavaScript array contains method in your projects.
JavaScript array
According to a survey by Stack Overflow, 63.61% of developers use JavaScript as their primary programming language. Arrays are powerful and versatile tools that can store and manipulate multiple values in a single variable. But how do you find out if an array contains a specific element?
Check if a JavaScript array contains a string
-1
. Let me show you an example:let colors = ["Red", "Green", "Blue"];
console.log(colors.indexOf("Green")); // Output: 1
console.log(colors.indexOf("White")); // Output: -1
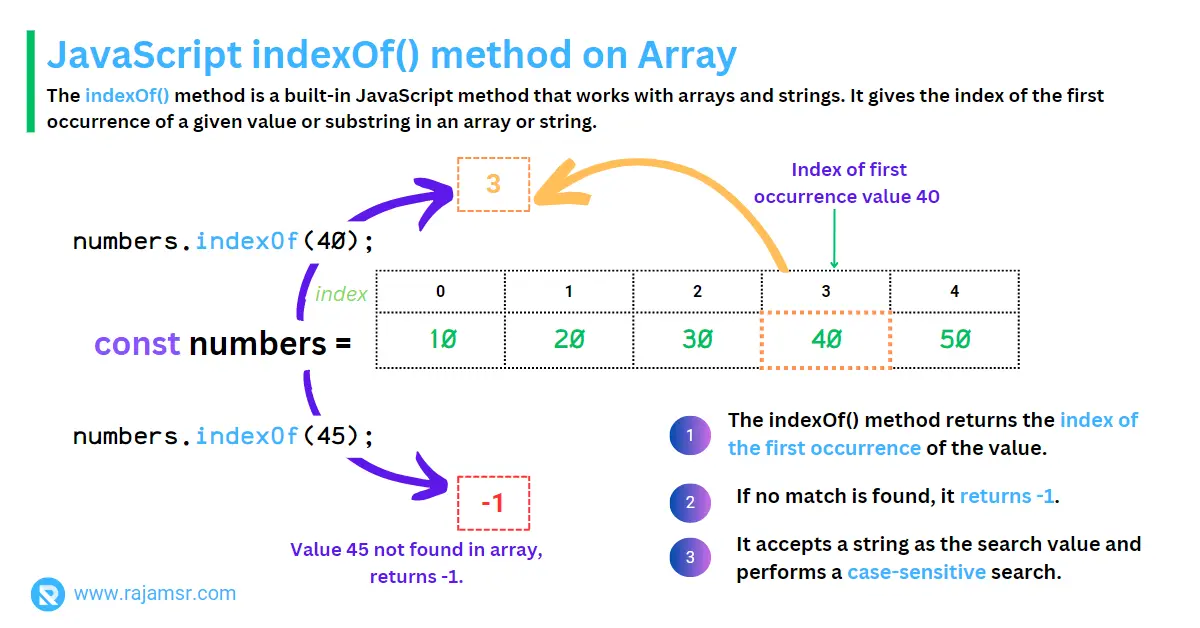
indexOf()
method is case-sensitive
, meaning that it will not match strings that have different capitalization. For example:let colors = ["Red", "Green", "Blue"];
console.log(colors.indexOf("green")); // Output: -1
indexOf()
to see if the word is in the array. Let me show you an example:let colors = ["Red", "Green", "Blue"];
console.log(colors.map(c=> c.toLowerCase()).indexOf("GREEN".toLowerCase()));
// Output: 1
find()
to loop through the array. Then you can use other awesome methods like JavaScript includes() or match() to see if the string is there. Let me show you an example:let colors = ["Red", "Green", "Blue"];
console.log(colors.some(c=>c.includes('Bl'))); // Output: true
console.log(colors.find(c=>c.match(/Bl/))); // Output: Blue
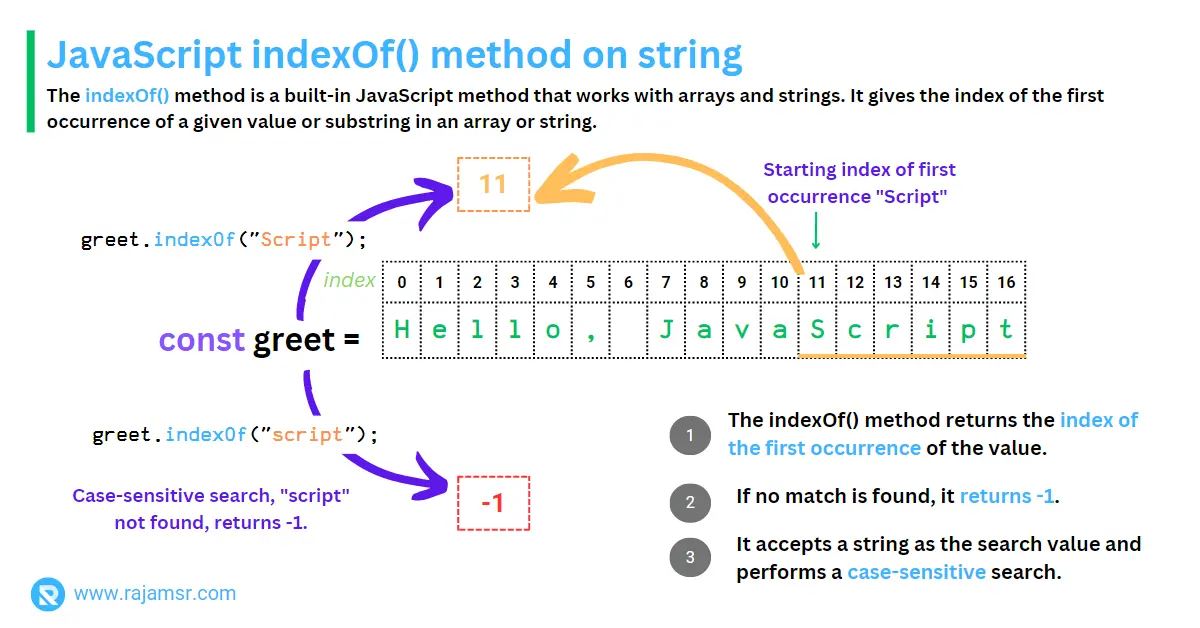
Check if a JavaScript array contains an object
JavaScript arrays are amazing, but they have a tricky feature. When you want to see if they have an object inside, you can’t just look at the object’s properties and values. You have to look at the object’s reference, which is like its address in memory. That means that two objects that look exactly the same are not equal, unless they point to the same place in memory. Let me show you an example:
let person1 = { name: "John", age: 30 };
let person2 = { name: "John", age: 30 };
let people = [person1, person2];
console.log(people.indexOf(person1)); // Output: 0
console.log(people.indexOf(person2)); // Output: 1
console.log(people.indexOf({ name: "John", age: 30 })); // Output: -1
JSON.stringify()
to turn them into strings and compare them. Like this:let person1 = { name: "John", age: 30 };
let person2 = { name: "John", age: 30 };
let person3 = { name: "John", age: 30 };
let people = [person1, person2, person3];
let objectToSearch = {
name: "John",
age: 30
};
console.log(people.find(person => JSON.stringify(person) === JSON.stringify(objectToSearch)));
// Output: {name: "John", age: 30}
Check if a JavaScript array contains a value
strict equality (===)
and loose equality (==)
. Strict means that the value and the type must be exactly the same. Loose means that JavaScript will try to make the values the same type before comparing them. For example: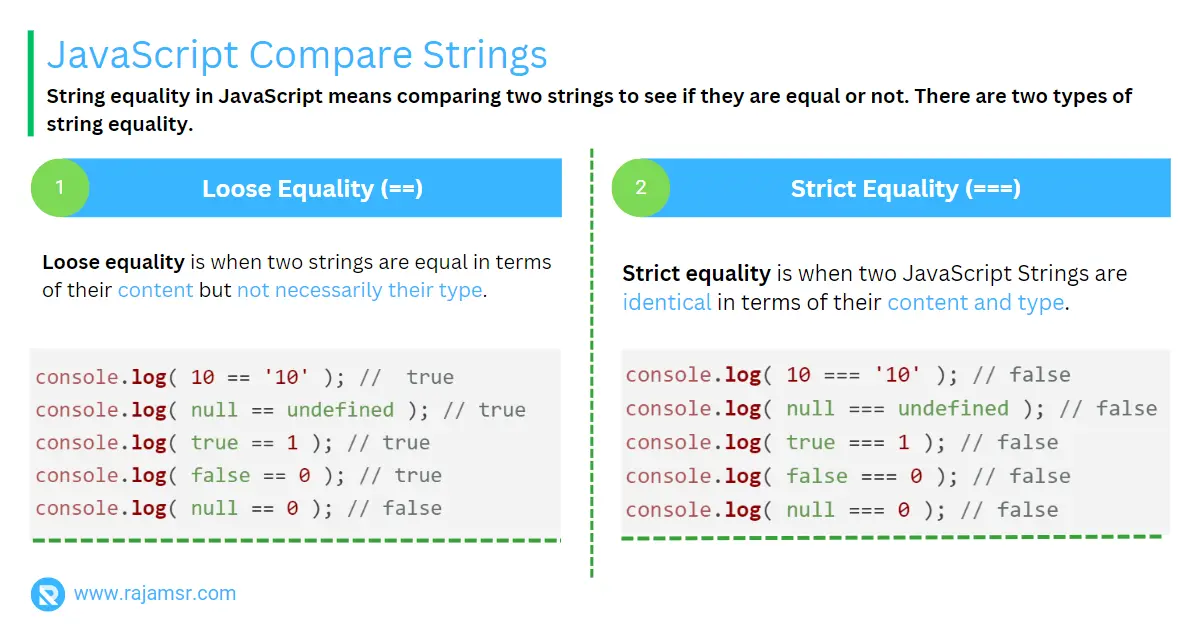
let numbers = [1, 2, 3, 4, 5];
console.log(numbers.indexOf(3)); // Output: 2
console.log(numbers.indexOf("3")); // Output: -1
console.log(numbers.indexOf(3) === numbers.indexOf("3")); // Output: false
console.log(numbers.indexOf(3) == numbers.indexOf("3")); // Output: false
indexOf()
method helps us find the position of a value in an array. But it has some rules. It only matches values that are exactly the same, not just similar. For example, it can tell the difference between a number and a string.Another rule is that the indexOf()
method does not like NaN
. NaN
means Not a Number, and it is a special value in JavaScript. The indexOf()
method cannot find NaN
in an array, because NaN
is not equal to anything, not even itself. So it always says -1, which means not found. But don’t worry, the indexOf()
method can work with other special values, like Infinity and -Infinity. They are equal to themselves, so the indexOf()
method can find them.let numbers = [1, 2, 3, NaN, Infinity, -Infinity];
console.log(numbers.indexOf(NaN)); // Output: -1
console.log(numbers.indexOf(Infinity)); // Output: 4
console.log(numbers.indexOf(-Infinity)); // Output: 5
Sometimes you don’t care about the type of a value, only if it matches another value. You can use some()
or find()
! These are methods that loop over the array and check each element. You can use the == operator to compare the element with the value. It will return true if they are equal in value, even if they have different types. Let me show you an example:
let numbers = [1, 2, 3, 4, 5];
console.log(numbers.some(number => number == true)); // Output: true
console.log(numbers.find(number => number == true)); // Output: 1
some()
or find()
to loop through the array. Then you can use Object.is()
to compare each element with the value. Object.is()
is like the ===
operator, but better. It knows that NaN
is equal to itself, and that +0
and -0
are different.Check if a JavaScript array contains a key
some()
or find()
. You can use two operators: JavaScript hasOwnProperty() or in. These operators let you see if the property exists in the object. Here is an example: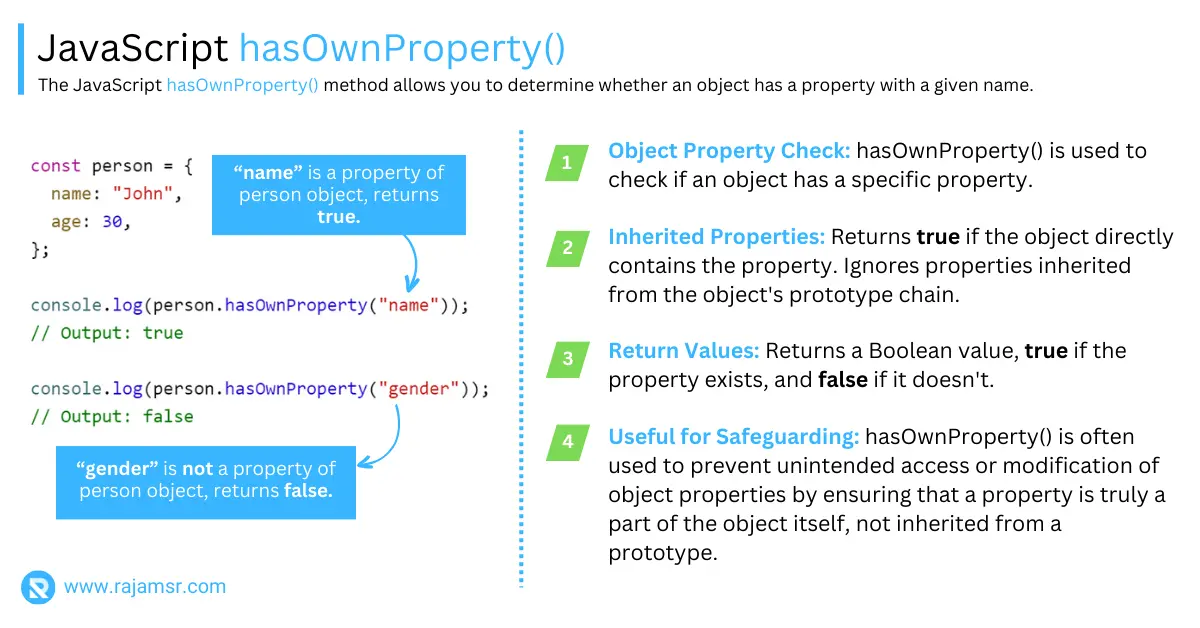
let person1 = { name: "Alice", age: 25, favoriteColor: "red" };
let person2 = { name: "Bob", age: 30, favoriteColor: "green" };
let person3 = { name: "Charlie", age: 35, favoriteColor: "blue" };
let people = [person1, person2, person3];
Let’s use this people array of objects in section.
console.log(people.some(person => person.hasOwnProperty("name")));
// Output: true
console.log(people.find(person => "age" in person));
// Output: {name: "Alice", age: 25, favoriteColor: "red"}
hasOwnProperty()
method only checks for the own properties of the object, meaning that it will not match keys that are inherited from the prototype chain. For example:console.log(people.some(person => person.hasOwnProperty("toString")));
// Output: false
Do you want to learn a cool trick with the in operator? It can do more than you think! It can check not only the properties that you assign to an object, but also the ones that it inherits from its prototype. Let me show you an example:
console.log(people.some(person => "toString" in person));
// Output: true
Sometimes you need to find out if an array has a certain key. But not any key. A key that belongs only to the array itself, not to its prototype. How can you do that? Easy! You can use a handy method called JavaScript Object.keys(). This method gives you a new array with all the own keys of the original array. Then you can use another method called indexOf()
to look for the key you want. Let me show you an example:
console.log(people.some(person => Object.keys(person).indexOf("toString") !== -1));
// Output: false
Check if a JavaScript array contains a boolean
Do you want to know if your JavaScript array has a true or false value in it? You can do that, but you have to be careful. Sometimes, JavaScript can change the type of your values when you compare them. This can lead to unexpected results. Let me show you an example:
let booleans = [true, false, true, false];
console.log(booleans.indexOf(true)); // Output: 0
console.log(booleans.indexOf("true")); // Output: -1
console.log(booleans.indexOf(true) === booleans.indexOf("true")); // Output: false
console.log(booleans.indexOf(true) == booleans.indexOf(true)); // Output: true
indexOf()
method work? It looks at each element in the array and checks if it is exactly the same as the boolean we are looking for. It does not care about the value, only the type. For example:let booleans = [true, false, true, false];
console.log(booleans.indexOf(1)); // Output: -1
console.log(booleans.indexOf(true)); // Output: 0
console.log(booleans.indexOf(1) === booleans.indexOf(true)); // Output: false
console.log(booleans.indexOf(1) == booleans.indexOf(true)); // Output: true
indexOf()
has some limitations. It does not work well with values that are not true or false, but can act like them. These values are called truthy
and falsy
values. Some examples of falsy values are 0, “”, null, undefined, and NaN. All other values are truthy. Here is an example to show you what I mean:let booleans = [true, false, true, false];
console.log(booleans.indexOf(0)); // Output: -1
console.log(booleans.indexOf(false)); // Output: 1
console.log(booleans.indexOf(0) === booleans.indexOf(false)); // Output: false
console.log(booleans.indexOf(0) == booleans.indexOf(false)); // Output: true
some()
or find()
to loop through the array and check each element with the loose equality operator. This way, you can match true with "true"
or false with "false"
. Here’s an example of how it works:let booleans = [true, false, true, false];
console.log(booleans.some(boolean => boolean == 1)); // Output: true
console.log(booleans.find(boolean => boolean == 1)); // Output: true
some()
and find()
that loop through the array for you. Then you can use the Boolean()
function to turn the elements into true or false values and compare them with the boolean you want. Here’s an example of how to do it:let booleans = [true, false, true, false];
console.log(booleans.some(boolean => Boolean(boolean) === false)); // Output: true
console.log(booleans.find(boolean => Boolean(boolean) === false)); // Output: false
Check if a JavaScript array contains a key-value pair
Imagine you have a list of people
, each with a name
and an age
. You want to find out if anyone in the list has a specific name and age, like Alice
and 25
. How can you do that?
You can use some handy method called some()
or find()
. They let you look through the list and check the name and age of each person. Then you can compare them with what you are looking for. Here is an example of how to do that:
let person1 = { name: "Alice", age: 25, favoriteColor: "red" };
let person2 = { name: "Bob", age: 30, favoriteColor: "green" };
let person3 = { name: "Charlie", age: 35, favoriteColor: "blue" };
let people = [person1, person2, person3];
We will use the above array of person objects for this section.
console.log(people.some(person => person.name === "Alice"
&& person.age === 25));
// Output: true
console.log(people.find(person => person["name"] === "Alice"
&& person["age"] === 25));
// Output: {name: "Alice", age: 25, favoriteColor:"red"}
Did you know that you can access the value of any property of an object in two different ways? That’s right, you can use either:
- The dot notation
- The bracket notation
They are both very useful, but there is a catch: the dot notation only works if the property name is a valid identifier. That means it cannot have any spaces, special characters, or reserved words. For example:
console.log(people.some(person => person.name === "Alice"
&& person.age === 25));
// Output: true
console.log(people.some(person => person.name === "Alice"
&& person.favoriteColor === "blue"));
// Output: true
console.log(people.some(person => person.name === "Alice"
&& person.Favorite Color === "blue"));
// returns SyntaxError: Unexpected identifier
The bracket notation allows you to use any string or expression as the property name, as long as it is enclosed in brackets. For example:
console.log(people.some(person => person["name"] === "Alice"
&& person["favorite color"] === "blue"));
// Output: false
String()
function to turn the value into a string first. Then you can use it as the property name. Here’s an example:console.log(people.some(person => person["name"] === "Alice"
&& person[String(3 + 2)] === "blue"));
// Output: false
Check if a JavaScript array contains another array
(x, y)
. Now, you want to find out if any of these points match a certain point, like [2, 3]
. How can you do that?One way is to use the some()
or find()
methods. These methods loop through the array and check each element. But how do you check if an element is an array and if it matches another array?You can use the Array.isArray()
method to see if an element is an array. Then, you can use the JavaScript every() or some()
methods to compare the elements of the two arrays. For example:let point1 = [1, 2];
let point2 = [2, 3];
let point3 = [3, 4];
let points = [point1, point2, point3];
let arrayToFind = [2, 3]
console.log(points.some(point => Array.isArray(point)
&& point.every((value, index) => value === arrayToFind[index])));
// Output: true
console.log(points.find(point => Array.isArray(point)
&& point.some((value, index) => value === arrayToFind[index])));
// returns [2, 3]
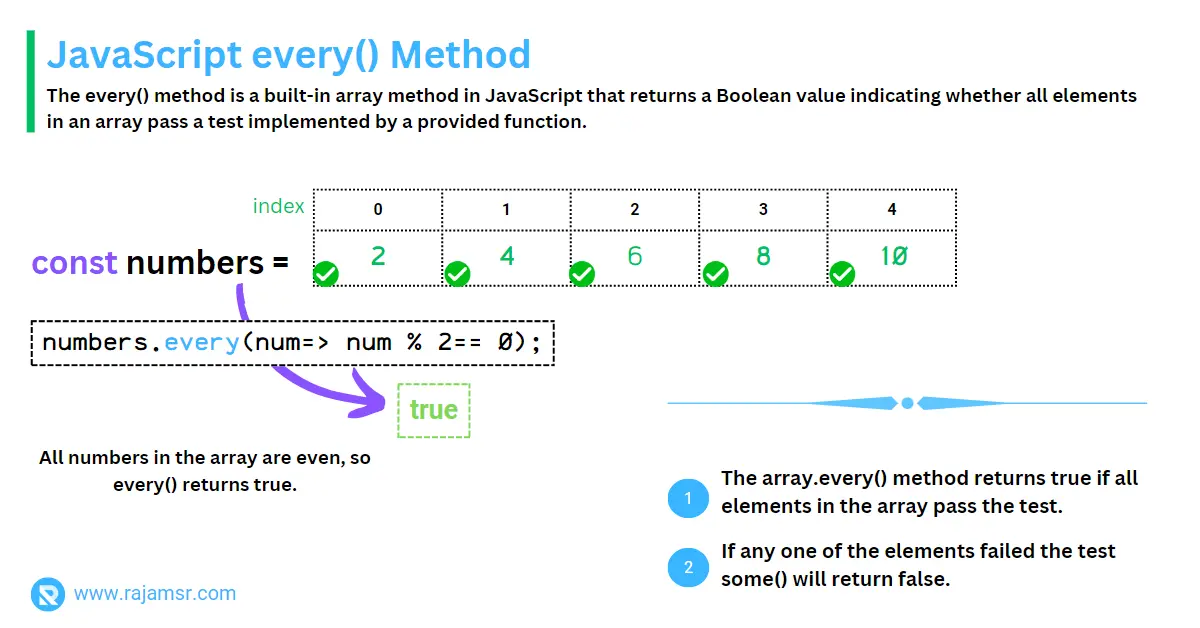
Array.isArray()
method only checks if the value is an array, not if it is an array of a certain length, type, or structure. For example:let point1 = [1, 2];
let point2 = [2, 3];
let point3 = [3, 4];
let points = [point1, point2, point3];
console.log(points.some(point => Array.isArray(point)
&& point.every((value, index) => value === [2, 3, 5][index])));
// Output: true
console.log( points.some(point => Array.isArray(point)
&& point.every((value, index) => value === [2][index])));
// Output: false
The every()
method lets you check if all the elements in an array meet a certain condition. But be careful, it won’t work if you want to compare arrays that have some matching elements, but not all. Here’s an example to show you what I mean:
let point1 = [1, 2];
let point2 = [2, 3];
let point3 = [3, 4];
let points = [point1, point2, point3];
console.log(points.some(point => Array.isArray(point)
&& point.every((value, index) => value === [2, 3][index])));
// Output: true
console.log(points.some(point => Array.isArray(point)
&& point.every((value, index) => value === [2, 4][index])));
// Output: false
some()
method. It’s a way to check if an array has at least one element that meets a certain condition. You can use some()
to compare two arrays and see if they have something in common. For example, look at this code:let point1 = [1, 2];
let point2 = [2, 3];
let point3 = [3, 4];
let points = [point1, point2, point3];
console.log(points.find(point => Array.isArray(point)
&& point.some((value, index) => value === [2, 3][index])));
// Output: [2, 3]
console.log(points.find(point => Array.isArray(point)
&& point.some((value, index) => value === [2, 4][index])));
// Output: [2, 3]
Object.is()
or JSON.stringify()
methods help you compare the content of the arrays. They check if the arrays have the same data type and structure. Here is an example of how to use them:let point1 = [1, 2];
let point2 = [2, 3];
let point3 = [3, 4];
let points = [point1, point2, point3];
let arrayToFind = [2, 3]
console.log(points.some(point => Array.isArray(point)
&& point.length === arrayToFind.length
&& point.every((value, index) => Object.is(value, arrayToFind[index]))));
// Output: true
console.log(points.some(point => Array.isArray(point)
&& point.length === arrayToFind.length
&& JSON.stringify(point) === JSON.stringify(arrayToFind)));
// Output: true
Conclusion
In this blog post, you learned how to use different methods and techniques to check if a JavaScript array contains a specific element.
You learned how to handle different elements, such as strings, objects, values, keys, booleans, and even other arrays.
You also learned how to overcome some of the limitations and challenges of these methods and techniques and write efficient and elegant code that can handle any array scenario.
I hope you found this blog post useful and informative. Over to you: Which method will you use to check if an array contains a certain element?