Have you ever encountered a situation where you need to compare or manipulate strings in JavaScript, but they have extra spaces at the beginning or end? If so, you are not alone. One of the common problems that many JavaScript developers face is how to remove extra spaces from the beginning and end of a string. This process is called JavaScript trim string. This way, you can ensure that your strings are consistent and accurate, and avoid any potential issues caused by extra spaces.
In this blog post, I will show you:
- How to trim strings in JavaScript using different methods
- JavaScript methods for trimming strings by length, character, or position
- Trimming strings in JavaScript: length, character, and position methods
- Different ways of trimming strings with JavaScript
- JavaScript trim string and add ellipsis (…)
JavaScript trim string methods
JavaScript provides three built-in methods for trimming strings: trim()
, trimStart()
, and trimEnd()
. These methods do not modify the original string, but return a new trimmed string instead.
- The
trim()
method strip any whitespace characters from both the beginning and the end of a string. Whitespace characters include spaces, tabs, newlines, and other Unicode characters that are considered as spaces. - The
trimStart()
method removes any whitespace characters from the beginning of a string. - The
trimEnd()
method removes any whitespace characters from the end of a string.
These methods do not accept any parameters, and they are easy to use. Here are some code examples of using these methods to trim strings:
// Using trim() to remove whitespace from both sides of a string
let name = " John Doe ";
console.log(name.trim());
// Output: "John Doe"
// Using trimStart() to remove whitespace from the beginning of a string
console.log(name.trimStart());
// Output: "John Doe "
// Using trimEnd() to remove whitespace from the end of a string
console.log(name.trimEnd());
// Output: " John Doe"
JavaScript trim string to length
Sometimes, you may want to trim a string to a specified length, rather than removing only whitespace characters.
For example, you may want to truncate a long string to fit a certain display area, or to create a summary of a longer text. In this case, you can use the JavaScript slice() method to trim a string to length.
The slice()
method extracts a part of a string and returns a new string. It accepts two parameters: the start index
and the end index
of the substring to be extracted.
- The start index is inclusive, while the end index is exclusive.
- If the end index is omitted, the method will extract the substring from the start index to the end of the string.
Here are some code examples of using slice() to trim strings to a length:
// Using slice() to trim a string to 10 characters
let message = "JavaScript runs everywhere on everything!";
console.log(message.slice(0, 10));
// Output: "JavaScript"
// Using slice() to trim a string from the 4th character to the end
console.log(message.slice(4));
// Output: "Script runs everywhere on everything!"
// Using slice() to trim a string from the 10th to the 20th character
console.log(message.slice(10, 20));
// Output: " runs ever"
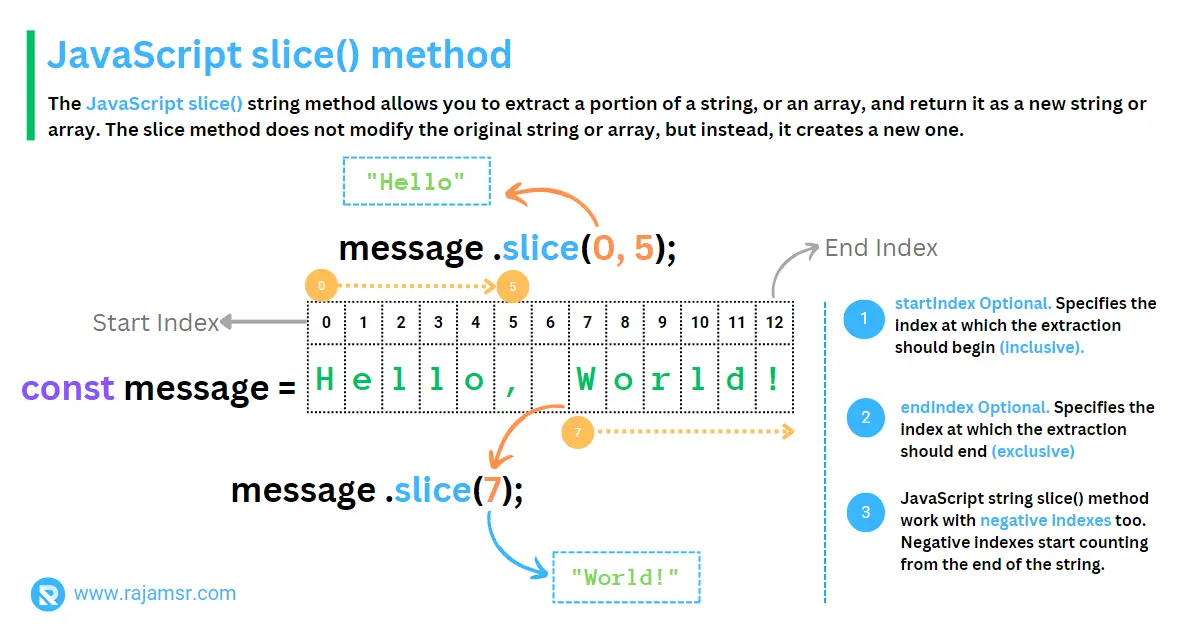
slice()
to trim strings to length has some advantages and disadvantages. Some of the advantages are:
Advantages | Disadvantages |
---|---|
|
|
JavaScript trim string to 10 characters
Sometimes, you may want to trim a string to a fixed length, such as 10 characters, regardless of its original length. To trim a string to 10 characters in JavaScript, you can use the slice()
method with a fixed index of 0 and 10. The slice()
method extracts a part of a string and returns a new string.
Here are some code examples of using JavaScript slice()
to trim strings to 10 characters:
// Using slice() to trim a string to 10 characters
let message = "JavaScript runs everywhere on everything!";
console.log(message.slice(0, 10));
// Output: "JavaScript"
// Using slice() to trim a string to 10 characters
let name = "Java";
console.log(name.slice(0, 10));
// Output: "Java"
// Using slice() to trim a string to 10 characters
let title = "Eloquent JavaScript";
console.log(title.slice(0, 10));
// Output: "Eloquent J"
slice()
method trims the string to 10 characters, regardless of its original length. However, this also means that it can cut off words or sentences in the middle, which may affect the readability or meaning of the string. So, you should use this method with caution and only when necessary.JavaScript trim string after character
Sometimes, you may want to trim a string after a specified character, such as a comma
, a dot
, or a space
. To trim a string after a character in JavaScript, you can use the JavaScript indexOf() and slice()
methods together.
The indexOf()
method returns the index of the first occurrence of a specified character in a string. If the character is not found, it returns -1
. The slice()
method, as we have seen before, extracts a part of a string and returns a new string.
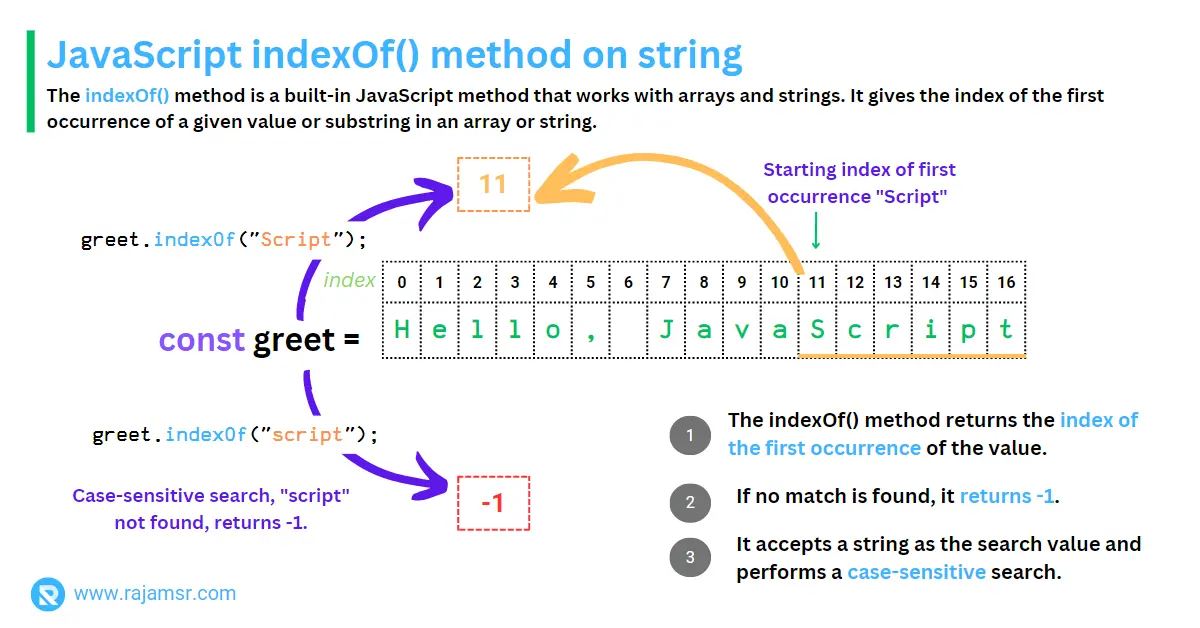
Here are some code examples of using indexOf()
and slice()
to trim strings after a character:
// Using indexOf() and slice() to trim a string after a comma
let address = "123 Main Street, New York, NY 10001";
console.log(address.slice(0, address.indexOf(",")));
// Output: "123 Main Street"
// Using indexOf() and slice() to trim a string after a dot
let email = "john.doe@example.com";
console.log(email.slice(0, email.indexOf(".")));
// Output: "john"
// Using indexOf() and slice() to trim a string after a space
let greeting = "Hello, world!";
console.log(greeting.slice(0, greeting.indexOf(" ")));
// Output: "Hello,"
As you can see, the indexOf()
and slice()
methods trim the string after the specified character, removing everything that follows it. However, this also means that it can lose some important or relevant information from the string. Therefore, you should use this method with care and only when required.
JavaScript comments were added to understand the functionality.
Trim string at character
Sometimes, you may want to trim a string at a specified character, such as a dash
, a colon
, or a slash
. This can be useful for splitting a string into two or more parts based on a certain delimiter or separator. To trim a string at a character in JavaScript, you can use the split() and join() methods together.
The split()
method splits a string into an array of substrings based on a specified character. It accepts one parameter: the character to use as the separator. The JavaScript join() method joins an array of substrings into a new string. It accepts one parameter: the character to use as the separator.
Here are some code examples for using split()
and join()
to trim strings at a character:
// Using split() and join() to trim a string at a dash
let title = "JavaScript-Trim-String";
console.log(title.split("-").join(" "));
// Output: "JavaScript Trim String"
// Using split() and join() to trim a string at a colon
let time = "06:56:08";
console.log(time.split(":").join("."));
// Output: "06.56.08"
// Using split() and join() to trim a string at a slash
let url = "https://www.bing.com\\search?q=javascript+trim+string";
console.log(url.split("\\").join("/"));
// Output: "https://www.bing.com/search?q=javascript+trim+string"
As you can see, the split()
and join()
methods trim the string at the specified character, replacing it with another character. However, this also means that it can change the format or structure of the string. Therefore, you should use this method with caution and only when essential.
JavaScript trim string from end
Sometimes, you may want to trim a string from the end, such as removing a suffix
, a punctuation mark
, or a whitespace character
. This can be useful for modifying or formatting a string according to your needs. For example, you may want to trim a string from the end to:
- Remove the file extension from a file name
- Remove the period from a sentence
- Remove the trailing space from a user input
To trim a string from the end, you can use the JavaScript string length property and the slice()
method together. The length
property returns the number of characters in a string.
Here are some code examples of using length and slice()
to trim strings from the end:
// Using length and slice() to trim a string from the end
let fileName = "Document.pdf";
console.log(fileName.slice(0, fileName.length - 4));
// Output: "Document"
// Using length and slice() to trim a string from the end
let sentence = "This is a sentence.";
console.log(sentence.slice(0, sentence.length - 1));
// Output: "This is a sentence"
// Using length and slice() to trim a string from the end
let userInput = "Hello ";
console.log(userInput.slice(0, userInput.length - 1));
// Output: "Hello"
As you can see, the length
and slice()
methods trim the string from the end, removing the specified number of characters. However, this also means that you need to know the exact number of characters to remove, which may not always be the case.
Trim string before character
Sometimes, you may want to trim a string before a specified character, such as a comma, a dot, or a space. This can be useful for removing unwanted or unnecessary parts of a string that precede a certain delimiter or separator.
To trim a string before a character in JavaScript, you can use the lastIndexOf()
and slice()
methods together. The lastIndexOf()
method returns the index of the last occurrence of a specified character in a string. If the character is not found, it returns -1
.
Here are some code examples of using the JavaScript array method lastIndexOf() and slice()
to trim strings before a character:
// Using lastIndexOf() and slice() to trim a string before a comma
let address = "123 Main Street, New York, NY 10001";
console.log(address.slice(address.lastIndexOf(",") + 1));
// Output: " NY 10001"
// Using lastIndexOf() and slice() to trim a string before a dot
let url = "https://www.bing.com/search?q=javascript+trim+string";
console.log(url.slice(url.lastIndexOf(".") + 1));
// Output: "com/search?q=javascript+trim+string"
// Using lastIndexOf() and slice() to trim a string before a space
let message = "Hello, JavaScript!";
console.log(message.slice(message.lastIndexOf(" ") + 1));
// Output: "JavaScript!"
lastIndexOf()
and slice()
methods trim the string before the specified character, removing everything that precedes it.Trim string add ellipsis (...)
Sometimes, you may want to truncate a string and add an ellipsis (…) at the end, such as when you want to create a summary, indicate truncation, or enhance aesthetics. An ellipsis is a punctuation mark that consists of three dots (...)
, and it is often used to show that something has been omitted or left out.
To trim a string and add an ellipsis at the end, you can use the slice()
and JavaScript string concatenation concat() methods together. The concat()
method concatenates two or more strings and returns a new string. It accepts one or more parameters.
Here are some code examples of using slice()
and concat()
to trim a string and add an ellipsis at the end:
// Using slice() and concat() to trim a string and add an ellipsis at the end
let message = "JavaScript runs everywhere on everything!";
console.log(message.slice(0, 20).concat("..."));
// Output: "JavaScript runs ever..."
console.log(message.slice(0, 30).concat("..."));
// Output: "JavaScript runs everywhere on ..."
As you can see, the slice()
and concat()
methods trim the string and add an ellipsis at the end, creating a new string. However, this also means that you need to specify the length of the substring to be extracted, which may not always be the case.
Trimming a string and adding an ellipsis at the end can have some applications, such as:
- Creating summaries: Trimming a string and adding an ellipsis can help you create a short and concise summary of a longer text, such as a blog post, a news article, or a book.
- Indicating truncation: Trimming a string and adding an ellipsis can help you indicate that a string has been truncated or cut off, such as when you want to fit a string into a limited display area or format.
- Enhancing aesthetics: Trimming a string and adding an ellipsis can help you enhance the aesthetics or appearance of a string, such as when you want to create a catchy headline, a slogan, or a quote.
Conclusion
In this blog post, I have shown you how to trim strings in JavaScript using different methods and techniques. I have explained how to trim spaces from a string using the trim()
method, and how to trim a string and add an ellipsis at the end using the slice()
and concat()
methods.
We have also discussed some implications and applications of trimming strings in JavaScript, such as improving readability, reducing memory usage, matching patterns, creating summaries, indicating truncation, or enhancing aesthetics.
Use the appropriate method for your purpose and situation. For example, use trim()
to trim spaces from both sides of a string, use slice()
and concat()
to trim a string and add an ellipsis at the end, or use other methods such as trimStart()
, trimEnd()
, indexOf()
, lastIndexOf()
, split()
, or join()
to trim a string in other ways.
Be careful not to lose or change the meaning or information of your string when trimming it. Add some indicators or explanations when trimming a string, especially when it is not obvious.
I hope this blog post has helped you learn how to trim strings in JavaScript. Over to you, how you are going to use JavaScript trim methods?